In Python, there are situations where you might need to verify whether a file exists.
Why is it important to check if a file exists?
Checking if a file exists is useful when you need to perform specific operations, such as opening, reading, or writing to the file.
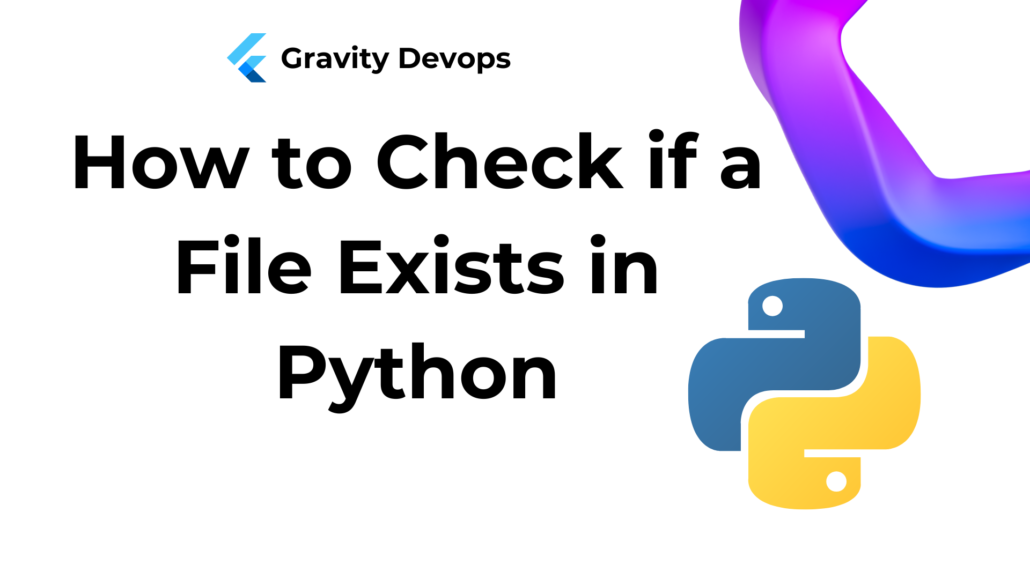
Attempting any of the above operations on a non-existent file can lead to errors and cause your program to crash.
To avoid crashes and ensure smooth operations, it’s a good practice to first check if a file exists at the specified path.
Fortunately, Python provides several built-in methods for checking if a file exists, such as using the os.path
and pathlib
modules.
With the os.path
module, you can specifically utilize:
- The
os.path.isfile(path)
method, which returnsTrue
if the specifiedpath
is a file or a symlink to a file. - The
os.path.exists(path)
method, which returnsTrue
if thepath
exists as a file, directory, or symlink.
With the pathlib
module, you can use the pathlib.Path(path).is_file()
function, which returns True
if the specified path
exists and is a file.
In this article, you’ll learn how to use Python to check if a file exists using the os.path
and pathlib
modules.
Let’s get started!
How to Check if a File Exists Using the os.path
Module
The os
module is a part of Python’s standard library (stdlib) and allows you to interact with and access the operating system.
The os
module provides functionalities that depend on the underlying operating system, such as creating and deleting files and directories, as well as copying and moving folder contents, among others.
Since the os
module is part of Python’s standard library, it comes pre-installed when you install Python on your system. You just need to import it at the beginning of your Python file using the import
statement:
import os
os.path
is a submodule of the os
module.
It provides two methods for working with files: isfile()
and exists()
, which return either True
or False
based on whether a file exists or not.
Since you’ll be using the os.path
submodule, you’ll need to import it at the beginning of your file, like this:
import os.path
How to Check if a File Exists Using the os.path.isfile()
Method in Python
The general syntax for the isfile()
method is as follows:
os.path.isfile(path)
The method takes a single argument, path
, which specifies the path to the file whose existence you want to check.
The path
argument is a string enclosed in quotation marks.
The isfile()
method returns a Boolean value—either True
or False
—based on whether the specified file exists.
Note that if the path points to a directory rather than a file, it will return False
.
Let’s look at an example of the method in action.
I want to check if the example.txt
file exists in my current working directory, python_project
.
The example.txt
file is in the same directory as my Python file, main.py
, so I’m using a relative file path.
I store the path to example.txt
in a variable called path
.
Next, I use the isfile()
method and pass path
as an argument to check if example.txt
exists at that location.
Since the file exists, the return value is True.
import os.path
path = './example.txt'
check_file = os.path.isfile(path)
print(check_file)
# output
# True
Alright, but what about absolute paths?
Here is the equivalent code using an absolute path. The example.txt
file is located within a python_project
directory, which is inside my home directory at /Users/dionysialemonaki/
.
import os.path
path = '/Users/dionysialemonaki/python_project/example.txt'
print(os.path.isfile(file_path))
# Output
# True
As mentioned earlier, the isfile()
method works only for files, not directories.
import os.path
path = '/Users/dionysialemonaki/python_project'
check_file = os.path.isfile(path)
print(check_file)
# output
# False
If the path ends with a directory, the return value will be False.
How to Check if a File Exists Using the os.path.exists()
Method in Python
The general syntax for the exists()
method is as follows:
os.path.exists(path)
The syntax above shows that the exists()
method is similar to the isfile()
method.
The os.path.exists()
method checks if the given path exists.
The key difference between exists()
and isfile()
is that exists()
returns True
if the specified path points to either a file or a folder, while isfile()
returns True
only if the path refers to a file, not a folder.
Note that if you lack the necessary access or permissions to the directory, exists()
will return False
, even if the path actually exists.
Let’s revisit the example from the previous section and use the exists()
method to check if the example.txt
file exists in the current working directory:
import os.path
path = './example.txt'
check_file = os.path.exists(path)
print(check_file)
# output
# True
Since the path to example.txt
exists, the output is True
.
As mentioned earlier, the exists()
method verifies whether the path to a directory or file is valid.
In the previous section, when I used the isfile()
method and the path pointed to a directory, the output was False
, even though the directory existed.
When using the exists()
method, if the path to a directory exists, the output will be True
:
import os.path
path = '/Users/dionysialemonaki/python_project'
check_file = os.path.exists(path)
print(check_file)
# output
# True
The exists()
method is useful when you want to check if a file or directory exists.
How to Check if a File Exists Using the pathlib
Module
The pathlib
module was introduced in Python 3.4.
Using the pathlib
module to check if a file exists is an object-oriented approach to handling filesystem paths.
Similar to the os.path
module mentioned earlier, you need to import the pathlib
module.
In particular, you must import the Path
class from the pathlib
module as follows:
from pathlib import Path
Next, create a new instance of the Path
class and initialize it with the file path you want to check.
from pathlib import Path
# create a Path object with the path to the file
path = Path('./example.txt')
You can use the type()
function to determine the data type.
from pathlib import Path
path = Path('./example.txt')
print(type(path))
# output is a pathlib object
# <class 'pathlib.PosixPath'>
This confirms that you have created a Path
object.
Let’s explore how to use the pathlib
module to check if a file exists using the is_file()
method, one of the built-in methods provided by the module.
How to Check if a File Exists Using the Path.is_file()
Method in Python
The is_file()
method checks whether a file exists.
It returns True
if the Path
object points to a file, and False
if the file doesn’t exist.
Here’s an example of how it works:
from pathlib import Path
# create a Path object with the path to the file
path = Path('./example.txt')
print(path.is_file())
# output
# True
Since the example.txt
file exists at the specified path, the is_file()
method returns True
.
Conclusion
In this article, you learned how to check if a file exists in Python using the os.path
and pathlib
modules, along with their respective methods.
Hopefully, you now understand the differences between the modules and know when to use each one.
Thank you for reading, and happy coding!