An array is a single variable that holds elements of different data types, allowing them to be accessed using one reference.
It is an ordered collection of values, where each value is called an element and is identified by an index.
Since these single variables contain a list of elements, you may want to process each element individually or perform various operations on them. This is where loops become useful.
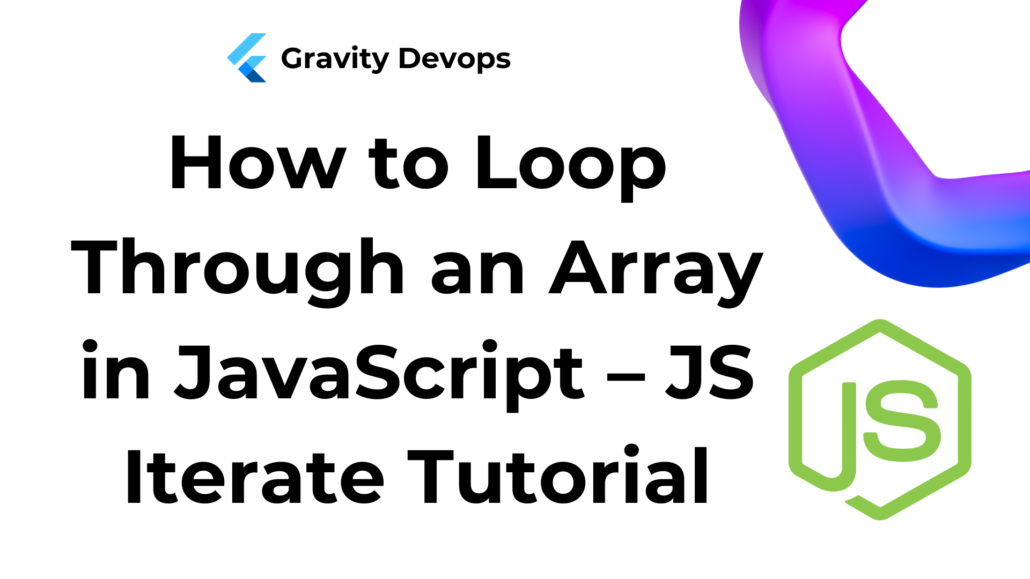
Here’s an interactive scrim about how to loop through an array in JavaScript:
What are Loops in JavaScript?
A loop is a programming construct that lets us repeat a specific operation a set number of times without having to write the same code repeatedly.
For example, if we have an array and want to print each element, instead of manually accessing each element by its index, we can use a loop to perform this operation in a single pass.
JavaScript offers several methods for looping through an array. In this article, we will cover the most commonly used ones, helping you learn different approaches and understand how they function.
For this article, we will use the following array:
const scores = [22, 54, 76, 92, 43, 33];
How to Loop Through an Array with a While Loop in JavaScript
You can use a while
loop to check a condition enclosed in parentheses ()
. If the condition is true
, the code inside the loop runs; if it is false
, the loop ends.
To loop through an array, we can use the length
property to ensure the loop continues until it reaches the last element of the array.
Now, let’s use the while
loop to iterate through the array:
let i = 0;
while (i < scores.length) {
console.log(scores[i]);
i++;
}
This will output each element of the array, one at a time:
22
54
76
92
43
33
In the loop above, we start by initializing the index at 0. Then, the index increments, outputting each element until the condition evaluates to false, signaling that we’ve reached the end of the array. When i = 6
, the condition stops being executed because the array’s last index is 5.
How to Loop Through an Array with a do…while
Loop in JavaScript
The do...while
loop is similar to the while
loop, but it executes the loop body first before checking the condition for subsequent iterations. This ensures that the loop’s body is always executed at least once.
Let’s use the do...while
loop to perform the same iteration and observe how it works:
let i = 0;
do {
console.log(scores[i]);
i++;
} while (i < scores.length);
This will display each element of the array:
22
54
76
92
43
33
As mentioned earlier, the loop will always execute once before evaluating the condition. For example, if we set the index i
to 6, and it is no longer less than scores.length
, the loop body will run first before the condition is checked.
let i = 6;
do {
console.log(scores[i]);
i++;
} while (i < scores.length);
This will return a single undefined
because there is no element at index 6 in the array. However, as you can see, it ran once before stopping.
How to Loop Through an Array with a for Loop in JavaScript
The for
loop is an iterative statement that evaluates specific conditions and repeatedly executes a block of code as long as those conditions remain true.
With the for
loop, we don’t need to initialize the index separately, as the initialization, condition, and iteration are all managed within the parentheses, as shown below:
for (let i = 0; i < scores.length; i++) {
console.log(scores[i]);
}
This will return all the elements, just like the other methods.
22
54
76
92
43
33
How to Loop Through an Array with a for…in
Loop in JavaScript
The for...in
loop provides a simpler way to iterate through arrays, as it gives us the key, which we can use to access the values in the array, like this:
for (i in scores) {
console.log(scores[i]);
}
This will display all the elements in the array:
22
54
76
92
43
33
How to Loop Through an Array with a for…of
Loop in JavaScript
The for...of
loop iterates over iterable objects like arrays, sets, maps, and strings. It has a similar syntax to the for...in
loop, but instead of accessing the key, it directly retrieves the element itself.
This is one of the simplest methods for looping through an array, introduced in JavaScript ES6.
for (score of scores) {
console.log(score);
}
This retrieves each element of the array directly, eliminating the need to use the index to access them.
22
54
76
92
43
33
How to Loop Through an Array with a forEach
Loop in JavaScript
The forEach()
array method loops through an array, executing a provided callback function once for each element in ascending index order.
This is a more advanced method that offers additional functionality beyond just looping through elements, but you can also use it for simple iteration like this:
scores.forEach((score) => {
console.log(score);
});
You can condense this into a single line like this:
scores.forEach((score) => console.log(score));
This will produce the same output as the previous methods:
22
54
76
92
43
33
Wrapping Up
In this article, we explored six different standard methods for looping through an array.
It’s important to understand all of these methods and then choose the one that works best for you, is cleaner to use, and is easier to read.