Terraform has gained immense popularity as a powerful infrastructure as code (IaC) tool for automating infrastructure deployment and management. As organizations move towards cloud-native solutions, Terraform expertise has become a highly sought-after skill for cloud engineers and DevOps professionals. If you’re preparing for a Terraform interview or simply looking to strengthen your knowledge, we’ve compiled 50 common Terraform interview questions and answers to help you get ready.
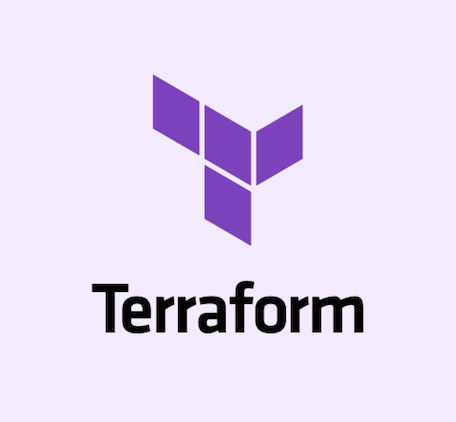
I. Terraform Basics:
- What is Terraform?
Terraform is an open-source IaC tool developed by HashiCorp, enabling users to define infrastructure configurations as code and automate resource provisioning across various cloud providers and on-premises infrastructure. - How does Terraform differ from traditional configuration management tools?
Unlike traditional configuration management tools (e.g., Puppet, Chef), Terraform focuses on provisioning and managing infrastructure resources, while configuration management tools are primarily concerned with the configuration of software and applications on those resources. - What are the key features of Terraform?
Terraform boasts several essential features, including infrastructure as code, declarative language, multi-cloud support, state management, plan and apply workflows, and modularity. - What is Terraform’s configuration file called, and what is its format?
Terraform uses files with a.tf
extension, and the configuration format is HashiCorp Configuration Language (HCL) or JSON. - What is the purpose of a Terraform state file?
The state file keeps track of the resources created and managed by Terraform. It helps Terraform understand the current state of the infrastructure and facilitates updates or destructions without recreating the entire infrastructure.
II. Terraform Configuration:
- How do you initialize a Terraform configuration?
You initialize a Terraform configuration in a directory using theterraform init
command. This downloads providers and initializes the working directory for Terraform usage. - What is a provider in Terraform?
A provider is a plugin that interacts with a specific cloud or infrastructure platform (e.g., AWS, Azure, GCP). Providers are responsible for managing the lifecycle of resources within that platform. - How do you define a provider in Terraform configuration?
You define a provider using theprovider
block in your Terraform configuration file. For example, to configure AWS as a provider, you’d use:
provider "aws" {
region = "us-west-2"
}
- What are variables in Terraform, and how are they defined?
Variables allow you to parameterize your Terraform configuration and make it more reusable. They can be defined in a separate.tf
file or as environment variables. - Explain data sources in Terraform.
Data sources enable you to query information from external resources (e.g., existing infrastructure) and use that data within your Terraform configuration. They do not create or manage resources; instead, they retrieve information for use. - How do you create resources in Terraform?
Resources are created using theresource
block. The block specifies the resource type and its attributes. For example, to create an AWS EC2 instance, you’d use:resource "aws_instance" "example" { ami = "ami-0c55b159cbfafe1f0" instance_type = "t2.micro" }
- What is a provisioner in Terraform?
Provisioners are used to execute scripts or commands on a resource after it’s created or updated. They help with tasks like configuration management and bootstrapping. - How can you manage multiple environments (e.g., development, production) in Terraform?
Terraform workspaces allow you to manage multiple environments within the same configuration directory. Each workspace maintains its own state and resources.- To create a new workspace:
terraform workspace new <workspace_name>
- To select a workspace:
terraform workspace select <workspace_name>
- To create a new workspace:
III. Terraform State and State Management:
- What is Terraform state locking?
Terraform state locking is a mechanism that prevents multiple users or processes from concurrently modifying the same Terraform state, reducing the risk of conflicts and data corruption. - Explain remote state in Terraform and why it’s beneficial.
Remote state involves storing the Terraform state in a shared and remote backend (e.g., AWS S3, Azure Blob Storage). This allows collaboration among team members and provides better state management and locking capabilities. - How do you configure remote state in Terraform?
Remote state is configured using theterraform
block within the configuration file. An example of configuring the AWS S3 backend:terraform { backend "s3" { bucket = "my-tf-state-bucket" key = "terraform.tfstate" region = "us-west-2" } }
- What happens if the Terraform state is lost? How can you recover it?
Losing the Terraform state can be catastrophic. To recover the state, if you have a remote backend, you can re-runterraform init
andterraform apply
to recreate the resources and update the state. If using local state, recovery becomes more challenging and might require manual intervention. - How can you manage sensitive data (e.g., passwords, API keys) in Terraform?
Terraform supports sensitive data management using input variables with sensitive flags, which prevent the data from being displayed in logs and outputs. Additionally, you can use Vault to manage secrets and encryption.
IV. Terraform Commands and Workflows:
- Explain the
terraform plan
command.terraform plan
examines the Terraform configuration and creates an execution plan, detailing what actions Terraform will take to achieve the desired state. It’s a safe way to preview changes before applying them. - What is the significance of the
-target
flag interraform plan
andterraform apply
?
The-target
flag allows you to specify a resource to be targeted for planning or applying, limiting the scope of operations to that specific resource. - How do you manage the order of resource creation or dependency in Terraform?
Terraform automatically determines the order of resource creation based on dependencies between resources. However, you can use thedepends_on
attribute to specify explicit dependencies when Terraform’s automatic ordering is insufficient. - Explain the
terraform apply
command.terraform apply
is used to apply the changes defined in the Terraform configuration. It creates or updates the infrastructure based on the desired state. - What happens if there’s an error during a
terraform apply
operation?
If an error occurs duringterraform apply
, Terraform will attempt to roll back changes to maintain the infrastructure’s desired state. It’s essential to handle errors gracefully and avoid partial deployments. - What is the purpose of the
-auto-approve
flag interraform apply
?
The-auto-approve
flag skips the interactive approval prompt during theterraform apply
operation, allowing for fully automated deployments. - How can you destroy resources created by Terraform?
Resources can be destroyed using theterraform destroy
command. This ensures that the infrastructure no longer exists, and the state is updated accordingly. - What is the purpose of the
-force
flag interraform destroy
?
The-force
flag allowsterraform destroy
to proceed without interactive approval, making it suitable for automated scripts or non-interactive environments.
V. Terraform Modules:
- What are Terraform modules?
Terraform modules are reusable components that encapsulate resources and configurations. They promote code reusability, maintainability, and consistency across multiple projects. - How do you create a Terraform module?
A Terraform module is defined within a directory containing.tf
files. You can pass input variables into the module and use them to parameterize the resources it creates. - How can you share a Terraform module with other team members or projects?
Terraform modules can be shared by publishing them to a version control system (e.g., GitHub) or a module registry (e.g., Terraform Registry). Others can then use the module by specifying its source in their configuration. - What is the purpose of the
count
parameter in a Terraform module?
Thecount
parameter enables resource duplication within a module, allowing you to create multiple instances of a resource without defining them explicitly. - What is the difference between
count
andfor_each
in Terraform?
Bothcount
andfor_each
are used to create multiple instances of resources, but the key difference is how they handle additions or removals from the configuration.count
creates resources based on the number specified, whilefor_each
maps resources to a set of keys and can dynamically add or remove resources based on the input.
VI. Terraform Best Practices and Troubleshooting:
- What are some best practices for writing Terraform configurations?
- Use version control to manage Terraform configurations.
- Leverage modules for reusability.
- Separate sensitive data from the configuration.
- Regularly backup Terraform state.
- How can you ensure Terraform configurations are secure?
To enhance security, limit access to Terraform state and ensure that sensitive data, such as credentials, is not exposed within the configuration. - What are some common issues faced while using Terraform?
Common issues include incorrect dependencies, conflicts with manual changes, and infrastructure drift. Terraform state locking can help mitigate these problems. - How can you manage infrastructure drift in Terraform?
Infrastructure drift occurs when manual changes are made to resources managed by Terraform. To manage drift, avoid making manual changes and use Terraform to make updates instead. - What is Terraform’s
taint
command used for?
Thetaint
command marks a resource as tainted, meaning it will be destroyed and recreated on the nextterraform apply
. It’s useful for troubleshooting or recreating resources with known issues. - What is Terraform’s
import
command used for?
Theimport
command allows you to import existing resources into Terraform state. It’s helpful when you start using Terraform for existing infrastructure.
VII. Terraform and Cloud Providers:
- How does Terraform manage cloud-specific resources like AWS S3 buckets or Azure virtual networks?
Terraform uses provider plugins to interact with cloud APIs, allowing it to create, update, and delete cloud-specific resources using the provider’s capabilities. - Can Terraform manage resources across multiple cloud providers simultaneously?
Yes, Terraform supports multi-cloud deployments, enabling users to manage resources across different cloud providers within the same configuration. - What is the purpose of Terraform’s
backend
configuration in cloud providers?
Thebackend
configuration defines where the Terraform state is stored. For cloud providers, this typically refers to remote storage (e.g., S3, Azure Blob Storage) for better collaboration and state management.
VIII. Terraform and Automation:
- How can you integrate Terraform with continuous integration/continuous deployment (CI/CD) pipelines?
Terraform can be integrated into CI/CD pipelines to automate infrastructure changes as part of the application deployment process. Tools like Jenkins, GitLab CI/CD, and AWS CodePipeline can execute Terraform commands in the pipeline. - What are Terraform workspaces, and how can you utilize them in a CI/CD environment?
Terraform workspaces allow multiple concurrent state environments within the same configuration. In a CI/CD environment, you can use workspaces to isolate different stages (e.g., development, staging, production).
IX. Terraform and Infrastructure Security:
- How can you ensure the security of Terraform configurations and state files?
- Encrypt Terraform state files using a remote backend.
- Limit access to Terraform state and configuration files.
- Store sensitive data securely, away from the configuration.
- What is least privilege in the context of Terraform and cloud providers?
Least privilege refers to providing the minimum required permissions to Terraform and other users or services, ensuring they only have access to necessary resources and actions.
X. Terraform Ecosystem:
- What is the Terraform Registry, and how can you use it?
The Terraform Registry is a public repository of Terraform modules and providers. You can use it to find and utilize existing modules for your infrastructure needs. - What is Terraform Cloud, and why would you use it?
Terraform Cloud is a SaaS offering by HashiCorp that provides collaborative features, remote state management, and CI/CD integration for Terraform projects. It simplifies collaboration and enhances state management in a team environment. - Explain Terraform Enterprise and its benefits.
Terraform Enterprise is a self-hosted version of Terraform Cloud, designed for larger organizations that require greater control and security. It offers additional features like private module registries and policy enforcement.
XI. Terraform and Other IaC Tools:
- How does Terraform compare to other IaC tools like CloudFormation or Ansible?
- Terraform is cloud-agnostic and supports multiple cloud providers.
- CloudFormation is AWS-specific, while Ansible is focused on configuration management.
- In what scenarios would you choose Terraform over other IaC tools?
- When working with multi-cloud or hybrid cloud environments.
- For infrastructure provisioning and management, rather than configuration management.
- What are the limitations of Terraform?
- Terraform does not handle configuration management like Ansible or Puppet.
- Certain cloud provider features might not be immediately available in Terraform, requiring manual configuration.
Terraform continues to shape the way modern infrastructure is managed, and its importance in the cloud computing landscape cannot be understated. As you prepare for a Terraform interview or seek to enhance your knowledge, reviewing these questions and answers will help solidify your understanding of Terraform’s core concepts and best practices. Good luck, and happy infrastructure provisioning!